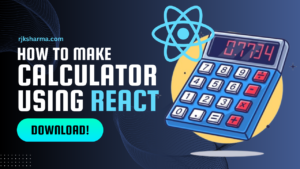
How to make Automatic Selfie Using Python | python project free source code
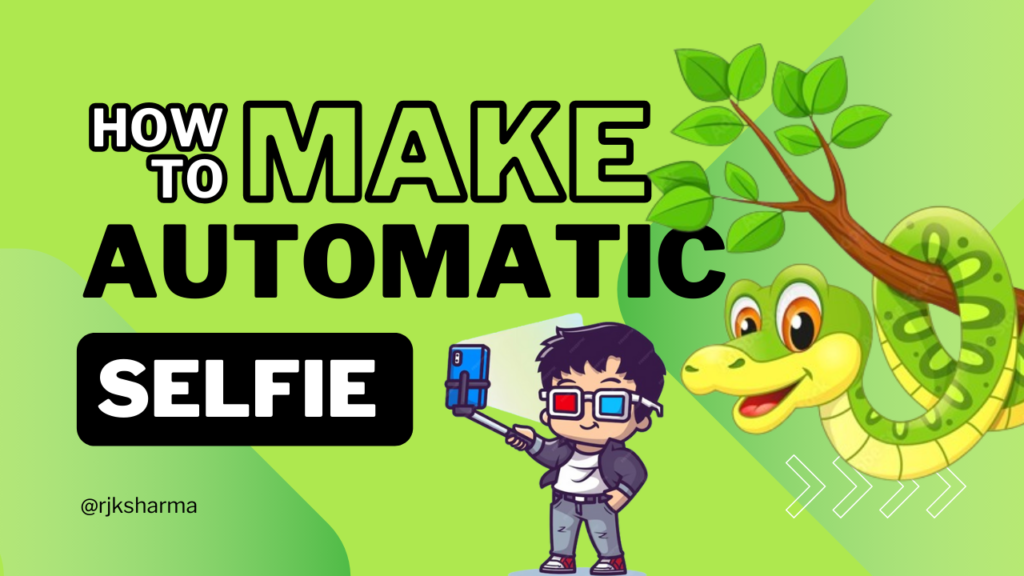
285 Downloads
Overview
Automating a selfie using Python involves utilizing the opencv-python library to access the computer’s webcam, capturing an image, and saving it automatically without user interaction. This process typically includes opening the webcam, capturing a frame, displaying it briefly on the screen, saving it as an image file (e.g., selfie.jpg), and then closing the webcam. The script handles these tasks sequentially, providing a simple yet effective way to take selfies programmatically using Python.
Features
- Library Used: Utilize opencv-python for accessing the webcam and capturing images.
- Capture Functionality: Use cv2.VideoCapture(0) to access the default webcam and cap.read() to capture a frame from it.
- Display and Save: Display the captured frame using cv2.imshow() and save it to a file using cv2.imwrite().
- User Interaction: Use cv2.waitKey() to display the captured image for a specific duration (e.g., 3 seconds) before saving it.
- Cleanup: Properly release the webcam using cap.release() and close all OpenCV windows with cv2.destroyAllWindows() after capturing and saving the selfie.
- Execution: Wrap the functionality in a function (take_selfie()) and execute it when the script is run (if __name__ == “__main__”).
Pre-requisites
-
- Access to Webcam: Make sure your computer has a webcam connected and that it’s functional. Most laptops have built-in webcams, and external webcams can be connected via USB.
- Basic Python Knowledge: Familiarity with basic Python programming concepts such as functions, control structures (like if-statements and loops), and how to run Python scripts from the command line or an IDE.
- Understanding of OpenCV Functions: While not mandatory, having a basic understanding of OpenCV functions such as cv2.VideoCapture(), cv2.imshow(), cv2.waitKey(), and cv2.imwrite() will help you customize and modify the script according to your needs.
- Python Installation: Ensure Python is installed on your system. You can download it from python.org and follow the installation instructions.
- OpenCV Library: Install the opencv-python library, which provides functions to work with images and video capture. You can install it using pip:bashCopy codepip install opencv-python.
Resources Required
- Python: Ensure Python is installed on your system.
- opencv-python: Install this library using pip install opencv-python to access webcam functionalities.
- Basic understanding of OpenCV: Familiarize yourself with basic OpenCV functions for capturing images from a webcam (cv2.VideoCapture, cv2.imshow, cv2.imwrite).
- Development Environment: Use a text editor or an integrated development environment (IDE) like PyCharm or VSCode to write and run your Python script.
Procedure
Install Required Library: Install opencv-python using pip if not already installed:
pip install opencv-python
- Write Python Script:
- Import the cv2 module for accessing webcam.
- Use cv2.VideoCapture(0) to open the default webcam.
- Capture a frame using cap.read().
- Display the frame using cv2.imshow() and wait for a few seconds using cv2.waitKey() to simulate the selfie-taking moment.
- Save the captured frame using cv2.imwrite() to a file like selfie.jpg.
- Release the webcam using cap.release() and close all OpenCV windows with cv2.destroyAllWindows().
- Run the Script: Execute the Python script to open the webcam, capture an image, display it momentarily, save it as selfie.jpg, and close the webcam.
- Adjustments: You may need to adjust camera settings or add additional features like face detection or filters based on OpenCV’s capabilities.
- Execution: After running the script, selfie.jpg will contain the captured image from the webcam, ready for viewing or further processing.
This procedure outlines the basic steps to implement automatic selfie capture using Python and OpenCV, providing a simple approach to interact with a webcam and save images programmatically.
Facing Difficultly to understand.
Watch this video to get more clear understanding to setup this project.